Maintaining a consistent coding style can be difficult in software development, especially in large or distributed teams. Different developers may have their preferences for indentation, variable naming, or code structure, leading to inconsistencies within the codebase. These inconsistencies can result in:
- Reduced Readability: Code becomes harder to understand and maintain.
- Merge Conflicts: Formatting differences cause unnecessary version control conflicts.
- Onboarding Challenges: New developers struggle to adapt to varying styles.
- Code Review Frustration: Code-style guarding comments waste time merging the code.
Solution: Code analyzers help enforce uniform coding standards automatically.
What is .editorconfig?
The .NET compiler platform (Roslyn) includes built-in analyzers that examine C# and Visual Basic code for style and quality issues. Starting from .NET 5, these analyzers are enabled by default. However, for projects targeting other .NET versions, such as .NET Core, .NET Standard, or .NET Framework, you must manually enable it by setting the EnableNETAnalyzers property to true.
.editorconfig allows you to configure and modify the behavior of .NET analyzers. You can use it to turn specific analyzers on or off, set severity levels (e.g., warning, error, suggestion), and fine-tune their rules to match your project’s coding standards.
Setting Up .editorconfig
Place a .editorconfig file in the root of your repository or solution.
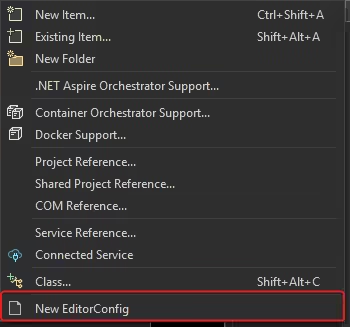
You’ll see a graphical editor when you open the .editorconfig file in Visual Studio.
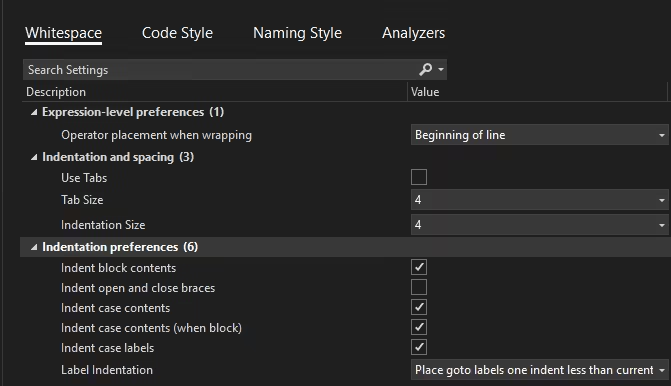
You can modify options for whitespace, code style, naming style, and analyzers.
The file source looks as follows:
# Remove the line below if you want to inherit .editorconfig settings from higher directories
root = true
# C# files
#### Core EditorConfig Options ####
# Indentation and spacing
indent_size = 4
indent_style = space
trim_trailing_whitespace = true
tab_width = 4
# New line preferences
end_of_line = crlf
insert_final_newline = false
.editorconfig files define formatting rules for specific source files or folders, with options grouped under section headers to specify their scope.
For example, [*.cs] selects all C# files with the .cs file extension within the current folder, including subfolders.
Severity Levels
.editorconfig allows you to define the severity of coding rule violations. The possible severity levels are:
- none → Disables the rule.
- suggestion → Provides a hint to improve code style.
- warning → Highlights a potential issue that should be reviewed.
- error → Enforces strict compliance and prevents compilation.
For example, you can force to use File Scoped namespaces by setting the severity level to error:
csharp_style_namespace_declarations = file_scoped:error
Using severity levels ensures consistent coding standards enforcement and helps teams avoid style-related issues during code reviews.
Best Practices
To maximize the benefits of .editorconfig, follow these best practices:
- Keep It at the Root: Place the .editorconfig file at the root of your repository to ensure all subdirectories inherit the settings.
- Use root = true: This prevents .editorconfig settings from being overridden by parent directories.
- Be Consistent: Align .editorconfig rules with team coding standards to avoid unnecessary changes.
- Use Meaningful Severity Levels: Set severity levels (suggestion, warning, error) based on your team’s coding guidelines.
- Update as Needed: Regularly review and adjust rules to reflect best practices and evolving team preferences.
Conclusion
Using .editorconfig in your .NET projects is a powerful way to maintain code consistency across your team. It reduces friction in code reviews and helps maintain a professional, uniform codebase. Regular review and updates of your .editorconfig settings ensure they continue to meet your team’s evolving needs.